Les traductions sont fournies par des outils de traduction automatique. En cas de conflit entre le contenu d'une traduction et celui de la version originale en anglais, la version anglaise prévaudra.
Interfaces de programmation compatibles avec DynamoDB
Chacun AWS SDK
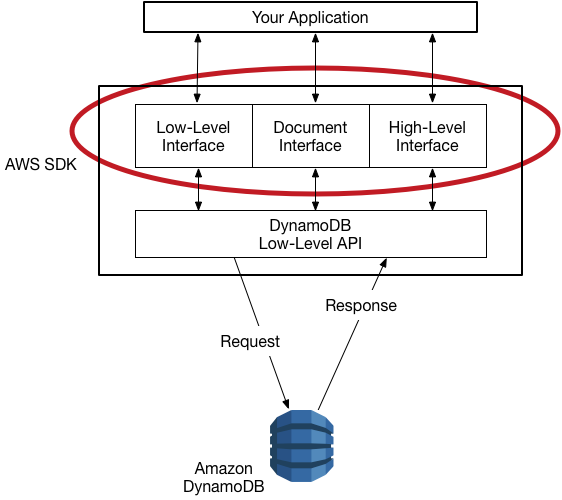
La section suivante met en évidence certaines des interfaces disponibles, en utilisant l' AWS SDK for Java en guise d'exemple (Toutes les interfaces ne sont pas toutes disponibles AWS SDKs.)
Rubriques
Interfaces de bas niveau compatibles avec DynamoDB
Chaque langue spécifique AWS SDK fournit une interface de bas niveau pour Amazon DynamoDB, avec des méthodes qui ressemblent étroitement aux requêtes DynamoDB de bas niveau. API
Dans certains cas, vous devrez identifier les types de données des attributs en utilisant des Descripteurs de type de données, tels que S
pour String (chaîne) ou N
pour Number (nombre).
Note
Une interface de bas niveau est disponible dans chaque langue AWS SDK spécifique.
Le programme Java suivant utilise l'interface de bas niveau de l' AWS SDK for Java.
import software.amazon.awssdk.regions.Region; import software.amazon.awssdk.services.dynamodb.model.DynamoDbException; import software.amazon.awssdk.services.dynamodb.DynamoDbClient; import software.amazon.awssdk.services.dynamodb.model.AttributeValue; import software.amazon.awssdk.services.dynamodb.model.GetItemRequest; import java.util.HashMap; import java.util.Map; import java.util.Set; /** * Before running this Java V2 code example, set up your development * environment, including your credentials. * * For more information, see the following documentation topic: * * https://docs.aws.amazon.com/sdk-for-java/latest/developer-guide/get-started.html * * To get an item from an Amazon DynamoDB table using the AWS SDK for Java V2, * its better practice to use the * Enhanced Client, see the EnhancedGetItem example. */ public class GetItem { public static void main(String[] args) { final String usage = """ Usage: <tableName> <key> <keyVal> Where: tableName - The Amazon DynamoDB table from which an item is retrieved (for example, Music3).\s key - The key used in the Amazon DynamoDB table (for example, Artist).\s keyval - The key value that represents the item to get (for example, Famous Band). """; if (args.length != 3) { System.out.println(usage); System.exit(1); } String tableName = args[0]; String key = args[1]; String keyVal = args[2]; System.out.format("Retrieving item \"%s\" from \"%s\"\n", keyVal, tableName); Region region = Region.US_EAST_1; DynamoDbClient ddb = DynamoDbClient.builder() .region(region) .build(); getDynamoDBItem(ddb, tableName, key, keyVal); ddb.close(); } public static void getDynamoDBItem(DynamoDbClient ddb, String tableName, String key, String keyVal) { HashMap<String, AttributeValue> keyToGet = new HashMap<>(); keyToGet.put(key, AttributeValue.builder() .s(keyVal) .build()); GetItemRequest request = GetItemRequest.builder() .key(keyToGet) .tableName(tableName) .build(); try { // If there is no matching item, GetItem does not return any data. Map<String, AttributeValue> returnedItem = ddb.getItem(request).item(); if (returnedItem.isEmpty()) System.out.format("No item found with the key %s!\n", key); else { Set<String> keys = returnedItem.keySet(); System.out.println("Amazon DynamoDB table attributes: \n"); for (String key1 : keys) { System.out.format("%s: %s\n", key1, returnedItem.get(key1).toString()); } } } catch (DynamoDbException e) { System.err.println(e.getMessage()); System.exit(1); } } }
Interfaces de documents compatibles avec DynamoDB
Beaucoup AWS SDKs proposent une interface documentaire qui vous permet d'effectuer des opérations de plan de données (création, lecture, mise à jour, suppression) sur les tables et les index. Avec une interface de document, vous n'avez pas besoin de spécifier Descripteurs de type de données. Les types de données découlent de la sémantique des données proprement dites. Ils fournissent AWS SDKs également des méthodes permettant de convertir facilement JSON des documents vers et depuis des types de données Amazon DynamoDB natifs.
Note
Les interfaces de document sont disponibles dans le AWS SDKs pour Java
Le programme Java suivant utilise l'interface de document de l' AWS SDK for Java. Le programme crée un objet Table
qui représente la table Music
, puis demande à cet objet d'utiliser GetItem
pour extraire une chanson. Le programme affiche ensuite l'année de sortie de la chanson.
La classe com.amazonaws.services.dynamodbv2.document.DynamoDB
implémente l'interface de document de DynamoDB. Observez la manière dont DynamoDB
agit en tant qu'encapsuleur autour du client de bas niveau (AmazonDynamoDB
).
package com.amazonaws.codesamples.gsg; import com.amazonaws.services.dynamodbv2.AmazonDynamoDB; import com.amazonaws.services.dynamodbv2.AmazonDynamoDBClientBuilder; import com.amazonaws.services.dynamodbv2.document.DynamoDB; import com.amazonaws.services.dynamodbv2.document.GetItemOutcome; import com.amazonaws.services.dynamodbv2.document.Table; public class MusicDocumentDemo { public static void main(String[] args) { AmazonDynamoDB client = AmazonDynamoDBClientBuilder.standard().build(); DynamoDB docClient = new DynamoDB(client); Table table = docClient.getTable("Music"); GetItemOutcome outcome = table.getItemOutcome( "Artist", "No One You Know", "SongTitle", "Call Me Today"); int year = outcome.getItem().getInt("Year"); System.out.println("The song was released in " + year); } }
Interfaces de persistance d'objets compatibles avec DynamoDB
Certains AWS SDKs proposent une interface de persistance des objets dans laquelle vous n'effectuez pas directement d'opérations sur le plan de données. Au lieu de cela, vous créez des objets représentant des éléments dans des tables et index Amazon DynamoDB, et interagissez uniquement avec ces objets. Cela vous permet d'écrire du code orienté objet plutôt que du code orienté base de données.
Note
Les interfaces de persistance des objets sont disponibles dans les AWS SDKs versions pour Java et. NET. Pour plus d'informations, consultez Interfaces de programmation de niveau supérieur pour DynamoDB pour DynamoDB.
import com.example.dynamodb.Customer; import software.amazon.awssdk.enhanced.dynamodb.DynamoDbEnhancedClient; import software.amazon.awssdk.enhanced.dynamodb.DynamoDbTable; import software.amazon.awssdk.enhanced.dynamodb.Key; import software.amazon.awssdk.enhanced.dynamodb.TableSchema; import software.amazon.awssdk.enhanced.dynamodb.model.GetItemEnhancedRequest; import software.amazon.awssdk.regions.Region; import software.amazon.awssdk.services.dynamodb.DynamoDbClient; import software.amazon.awssdk.services.dynamodb.model.DynamoDbException;
import com.example.dynamodb.Customer; import software.amazon.awssdk.enhanced.dynamodb.DynamoDbEnhancedClient; import software.amazon.awssdk.enhanced.dynamodb.DynamoDbTable; import software.amazon.awssdk.enhanced.dynamodb.Key; import software.amazon.awssdk.enhanced.dynamodb.TableSchema; import software.amazon.awssdk.enhanced.dynamodb.model.GetItemEnhancedRequest; import software.amazon.awssdk.regions.Region; import software.amazon.awssdk.services.dynamodb.DynamoDbClient; import software.amazon.awssdk.services.dynamodb.model.DynamoDbException; /* * Before running this code example, create an Amazon DynamoDB table named Customer with these columns: * - id - the id of the record that is the key. Be sure one of the id values is `id101` * - custName - the customer name * - email - the email value * - registrationDate - an instant value when the item was added to the table. These values * need to be in the form of `YYYY-MM-DDTHH:mm:ssZ`, such as 2022-07-11T00:00:00Z * * Also, ensure that you have set up your development environment, including your credentials. * * For information, see this documentation topic: * * https://docs.aws.amazon.com/sdk-for-java/latest/developer-guide/get-started.html */ public class EnhancedGetItem { public static void main(String[] args) { Region region = Region.US_EAST_1; DynamoDbClient ddb = DynamoDbClient.builder() .region(region) .build(); DynamoDbEnhancedClient enhancedClient = DynamoDbEnhancedClient.builder() .dynamoDbClient(ddb) .build(); getItem(enhancedClient); ddb.close(); } public static String getItem(DynamoDbEnhancedClient enhancedClient) { Customer result = null; try { DynamoDbTable<Customer> table = enhancedClient.table("Customer", TableSchema.fromBean(Customer.class)); Key key = Key.builder() .partitionValue("id101").sortValue("tred@noserver.com") .build(); // Get the item by using the key. result = table.getItem( (GetItemEnhancedRequest.Builder requestBuilder) -> requestBuilder.key(key)); System.out.println("******* The description value is " + result.getCustName()); } catch (DynamoDbException e) { System.err.println(e.getMessage()); System.exit(1); } return result.getCustName(); } }